Getting Started with Next.js: A Comprehensive Guide
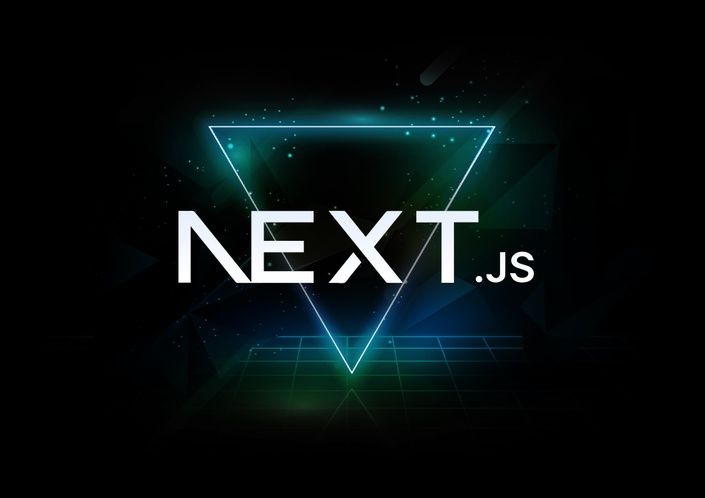
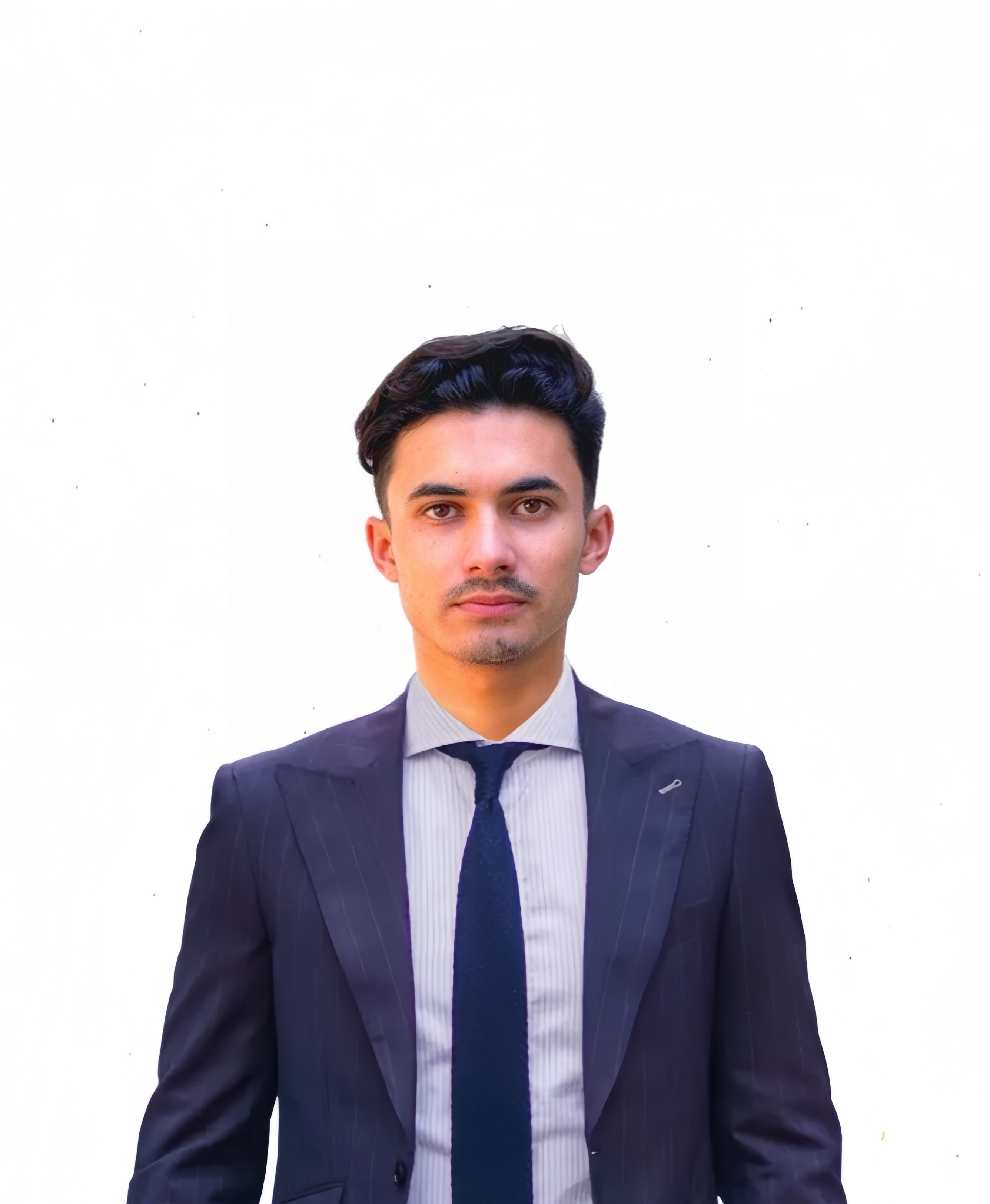
A full Stack web developer with over 5 years of experience
Getting Started with Next.js: A Comprehensive Guide
Next.js is a powerful React framework that enables developers to build server-rendered applications with ease. Whether you are a beginner or an experienced developer, Next.js provides features like automatic static optimization, API routes, and seamless deployment. In this guide, we will walk you through setting up your first Next.js project and exploring its core features.
Why Choose Next.js?
Next.js offers several advantages, making it a popular choice among developers:
- Hybrid Rendering: Supports both static and server-side rendering (SSR).
- Automatic Code Splitting: Optimizes performance by loading only necessary code.
- API Routes: Built-in backend functionality without needing an external server.
- SEO-Friendly: Pre-renders pages for better search engine indexing.
- Fast Refresh: Instant updates during development.
Setting Up Your Next.js Project
To start a new Next.js project, follow these steps:
1. Install Node.js
Ensure you have Node.js installed on your system. You can check the version by running:
node -v
2. Create a New Next.js App
You can quickly bootstrap a Next.js application using create-next-app
:
npx create-next-app@latest my-nextjs-app
cd my-nextjs-app
Alternatively, using yarn:
yarn create next-app my-nextjs-app
3. Start the Development Server
Run the following command to start your app in development mode:
npm run dev
By default, your Next.js app will be available at http://localhost:3000/
.
Understanding the Folder Structure
A newly created Next.js project includes the following structure:
my-nextjs-app/
|-- pages/ # Contains application routes (React components)
|-- public/ # Stores static assets like images
|-- styles/ # Stores CSS styles
|-- package.json # Project dependencies and scripts
|-- next.config.js # Custom Next.js configuration (optional)
Creating Pages in Next.js
Next.js follows a file-based routing system. Any React component inside the pages/
directory automatically becomes a route.
Example: Creating a New Page
Create a new file inside pages/
called about.js
:
export default function About() {
return (
<div>
<h1>About Us</h1>
<p>Welcome to the About page of our Next.js app.</p>
</div>
);
}
Now, navigating to http://localhost:3000/about
will display this page.
Adding API Routes
Next.js allows you to create API endpoints inside the pages/api/
directory.
Example: Creating an API Route
Create a new file pages/api/hello.js
:
export default function handler(req, res) {
res.status(200).json({ message: "Hello, Next.js!" });
}
Visiting http://localhost:3000/api/hello
will return a JSON response.
Deploying Your Next.js App
Deploying a Next.js app is straightforward with platforms like Vercel and Netlify.
Deploying on Vercel
- Install the Vercel CLI:
npm install -g vercel
- Run
vercel
inside your project directory and follow the instructions.
Conclusion
Next.js is a versatile framework that simplifies React development with built-in optimizations. By following this guide, you should have a basic understanding of how to set up a Next.js project, create pages, add API routes, and deploy your application.
Ready to take your Next.js skills to the next level? Explore more advanced features like dynamic routing, middleware, and server-side data fetching!
Was this article helpful?